Sometimes, we can use the wizard to allow the user to select the parameter and download the report result to the Excel.
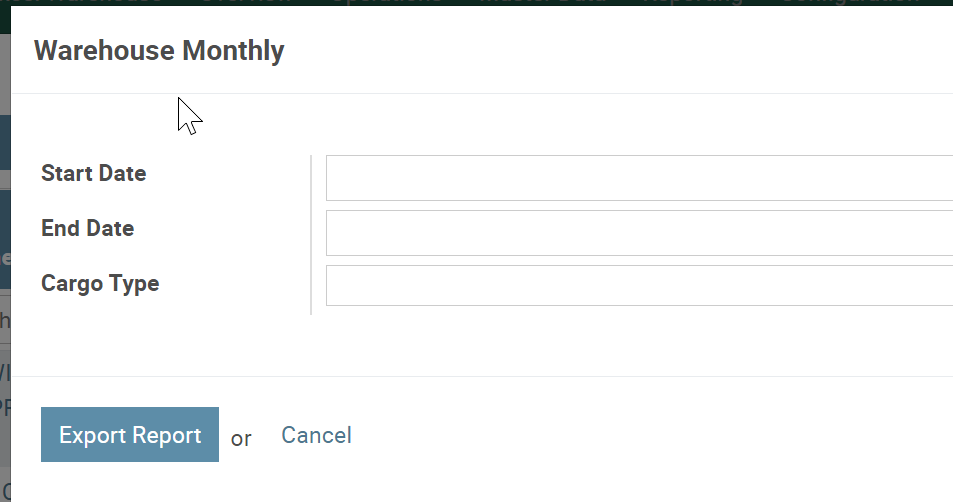
Start by creating the wizard.
The wizard XML.
<record id="view_warehouse_monthly_form" model="ir.ui.view">
<field name="name">view.warehouse.monthly.form</field>
<field name="model">warehouse.report</field>
<field name="arch" type="xml">
<form string="Warehouse Monthly Report">
<group>
<field name="date_from"/>
<field name="date_to"/>
<field name="cargo_type"/>
</group>
<footer>
<button string="Export Report" name="export_report" type="object" class="oe_highlight"/>
or
<button string="Cancel" class="oe_link" special="cancel" />
</footer>
</form>
</field>
</record>
Implement the export_report method to search for the result and generate your own excel report name , as well as pass the data to the Excel Report.
class WarehouseReport(models.TransientModel):
_name = "warehouse.report"
date_from = fields.Date(string='Start Date',)
date_to = fields.Date(string='End Date',)
cargo_type = fields.Selection([('fcl', 'FCL'), ('lcl', 'LCL')], string='Cargo Type')
@api.multi
def export_report(self):
self.ensure_one()
domain = [('receipt_date', '>=', self.date_from),
('receipt_date', '<=', self.date_to),
('job_status', 'not in', ['04', '01'])]
if self.cargo_type:
domain.append(('cargo_type', '=', self.cargo_type))
jobsheets = self.env['warehouse.tally.sheet'].search(domain, order="receipt_date asc")
if jobsheets:
mylist = []
for jobsheet in jobsheets:
mylist.append(jobsheet.id)
report_name = 'Warehouse Monthly - ' + str(self.date_from) + '.xlsx'
self.env.ref('stock_card_report.action_warehouse_report_xlsx').report_file = report_name
return self.env.ref('stock_card_report.action_warehouse_report_xlsx').report_action(self,
data={'mylist': mylist,
'date_from': self.date_from.strftime("%d-%m-%Y"),
'date_to': self.date_to.strftime("%d-%m-%Y"),
})
Declare the report in the xml.
<?xml version="1.0" encoding="utf-8"?>
<odoo>
<report id="action_warehouse_report_xlsx"
string="Warehouse Report"
model="warehouse.report"
report_type="xlsx"
name="my_module.report_warehouse_xlsx"
menu="False"
file = "my_module.report_warehouse_xlsx"
/>
</odoo>
Inherit the report.report_xlsx.abstract and implement the generate_xlsx_report.
Loop through the data parameter to populate the excel.
class WarehouseReportXlsx(models.AbstractModel):
_name = 'report.my_module.report_warehouse_xlsx'
_inherit = 'report.report_xlsx.abstract'
def generate_xlsx_report(self, workbook, data, lines):
sheet = workbook.add_worksheet('Warehouse Report')
format_header = workbook.add_format({'align': 'left', 'valign': 'vcenter','font_size': 14, 'bold': True})
format_header.set_font_color('blue')
sheet.write(0, 0, 'Warehouse Monthly Report', format_header)
can you share the code